Here are the results from VS 2017 Output, and a screenshot of the errors:
1>------ Rebuild All started: Project: HelloWorld.vs2017, Configuration: Debug x64 ------
1> HelloWorld.vs2017 -> C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld\bin\x64\Debug\HelloWorld.exe
2>------ Rebuild All started: Project: HelloWorld_CUDA.vs2017, Configuration: Debug x64 ------
2>
2>C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld_CUDA>"C:\Program Files\NVIDIA GPU Computing Toolkit\CUDA\v9.1\bin\nvcc.exe" -ccbin "C:\Program Files (x86)\Microsoft Visual Studio 14.0\VC\bin\x86_amd64" -x cu -I"C:\Program Files\NVIDIA GPU Computing Toolkit\CUDA\v9.1\include" -I"C:\Program Files\NVIDIA GPU Computing Toolkit\CUDA\v9.1\include" -G --keep-dir x64\Debug -maxrregcount=0 --machine 64 --compile -g -DWIN32 -DWIN64 -D_DEBUG -D_CONSOLE -D_WINDLL -D_MBCS -Xcompiler "/EHsc /W3 /nologo /Od /FS /Zi /RTC1 /MDd " -o x64\Debug\hybridizer.wrappers.cu.obj "C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld_CUDA\hybridizer-generated-sources\hybridizer.wrappers.cu" -clean
2>hybridizer.wrappers.cu
2>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\V140\Microsoft.CppBuild.targets(368,5): warning MSB8004: Output Directory does not end with a trailing slash. This build instance will add the slash as it is required to allow proper evaluation of the Output Directory.
2>HYBRIDIZER -- Build status file missing, generating
2>Build satellite full path: C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\bin\Hybridizer.VSIntegration.BuildSatellite.exe
2>Hybridizer binary full path: C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\bin\Hybridizer.exe
2>Satellite working directory: C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld_CUDA\hybridizer-generated-sources
2>Builtins: C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\include\hybridizer.cuda.builtins
2>Hybridizer out: [INFO] : entering BuildSatellite program
2>Hybridizer out: [INFO] : Loaded assembly from C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld\bin\x64\Debug\HelloWorld.exe
2>Hybridizer out: [INFO] : Found type <HelloWorld.Program> with <1> methods
2>Hybridizer out: [INFO] : Done searching assembly for hybridizer directives
2>Hybridizer out: Hybridizer full path: C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\bin\Hybridizer.exe
2>Hybridizer out: [INFO] : found type Altimesh.Hybridizer.Application.Program
2>Hybridizer out: [INFO] : found method Void Main(System.String[])
2>Hybridizer out: 2018/02/04 16:50:47:516 [INFO] Hybridizer-BASIC (c) Altimesh 2011-2016 - 1.0.0.0
2>Hybridizer out: 2018/02/04 16:50:48:032 [INFO] Hybridizer license: Trial version - for evaluation purpose only
2>Hybridizer out: 2018/02/04 16:50:48:329 [INFO] Processing assembly C:\xxxx\GitHub\hybridizer-basic-samples\HybridizerBasicSamples_CUDA91\1.Simple\HelloWorld\HelloWorld\bin\x64\Debug\HelloWorld.exe
2>Hybridizer out: 2018/02/04 16:50:48:344 [WARN] WARNING : using default builtins
2>Hybridizer out: 2018/02/04 16:50:48:376 [INFO] Loaded built-in configuration from C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\include\hybridizer.cuda.builtins
2>Hybridizer out: 2018/02/04 16:50:48:376 [INFO] Processing type HelloWorld.Program
2>Hybridizer out: 2018/02/04 16:50:48:423 [INFO] Reading for method Void Run(Int32, Double[], Double[]) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:516 [INFO] Registering methods
2>Hybridizer out: 2018/02/04 16:50:48:516 [INFO] Reading for method Void Run(Int32, Double[], Double[]) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:532 [INFO] Reading for method Void Run(Int32, Double[], Double[]) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:563 [INFO] Reading for method Void Run(Int32, Double[], Double[]) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:673 [INFO] Reading for method Void .ctor() from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:673 [INFO] No symbols found for Void .ctor()
2>Hybridizer out: 2018/02/04 16:50:48:673 [INFO] Reading for method Void .ctor() from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:673 [INFO] No symbols found for Void .ctor()
2>Hybridizer out: 2018/02/04 16:50:48:719 [INFO] Reading for method Void b__0(Int32) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:719 [INFO] Reading for method Void b__0(Int32) from assembly HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null
2>Hybridizer out: 2018/02/04 16:50:48:766 [INFO] Reading for method System.Threading.Tasks.ParallelLoopResult For(Int32, Int32, System.Action1[System.Int32]) from assembly mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089 2>Hybridizer out: 2018/02/04 16:50:48:923 [INFO] No symbols found for System.Threading.Tasks.ParallelLoopResult For(Int32, Int32, System.Action
1[System.Int32])
2>Hybridizer out: 2018/02/04 16:50:48:938 [INFO] Flavor CUDA has 4 methods
2>Hybridizer out: 2018/02/04 16:50:48:969 [INFO] Flavor CUDA has 4 methods
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] Listing types found for flavor
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.Object> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <HelloWorld.Program>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <HelloWorld.Program+<>c__DisplayClass0_0>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.Threading.Tasks.Parallel>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.ValueType>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <HelloWorld.Program> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <HelloWorld.Program+<>c__DisplayClass0_0>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.Double[]> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <HelloWorld.Program+<>c__DisplayClass0_0>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.ValueType> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.Threading.Tasks.ParallelLoopResult>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.Boolean> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.Threading.Tasks.ParallelLoopResult>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.Byte> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.Threading.Tasks.ParallelLoopResult>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] found type <System.Nullable`1[[System.Int64, mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089]]> used by :
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] <System.Threading.Tasks.ParallelLoopResult>
2>Hybridizer out: 2018/02/04 16:50:48:985 [INFO] Done listing types found for flavor
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en-US, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en-US, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en, PublicKeyToken=null
2>Hybridizer out: Not Found mscorlib.XmlSerializers, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089
2>Hybridizer out: 2018/02/04 16:50:49:376 [WARN] [WARNING] Non blittable value type HelloWorld.Program+<>c__DisplayClass0_0 may have poor marshalling performance
2>Hybridizer out: 2018/02/04 16:50:49:376 [WARN] [WARNING] Non blittable value type System.Threading.Tasks.ParallelLoopResult may have poor marshalling performance
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en-US, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en-US, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en, PublicKeyToken=null
2>Hybridizer out: Not Found Altimesh.Hybridizer.Application.resources, Version=1.0.0.0, Culture=en, PublicKeyToken=null
2>Hybridizer out: [INFO] : PTXJitter called <"C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\bin\Hybridizer.PTXJitterService.9.1.exe" 00000000000004A8 0000000000000568>
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: ======================= ERROR in Hybridizer JIT ==========================
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: [ERROR] : System.Reflection.TargetInvocationException: Exception has been thrown by the target of an invocation. ---> Transcoder.Disassembly.UserReportedException: HYBRIDIZER - INTERNAL ERROR
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at Altimesh.Hybridizer.Application.Toolkit.Run(String[] args)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at Altimesh.Hybridizer.Application.Program.Main(String[] args)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: --- End of inner exception stack trace ---
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at System.RuntimeMethodHandle.InvokeMethod(Object target, Object[] arguments, Signature sig, Boolean constructor)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at System.Reflection.RuntimeMethodInfo.UnsafeInvokeInternal(Object obj, Object[] parameters, Object[] arguments)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at System.Reflection.RuntimeMethodInfo.Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: at Transcoder.CudaDotNet.CLRHostStarter.AppDomainStarter.RunContent(String args)
2>C:\Users\xxxx.xxxx\AppData\Local\Microsoft\VisualStudio\15.0_775c7353\Extensions\rcr1wcx4.bjz\vsintegration\Hybridizer.targets(80,13): error : Hybridizer error: [FATAL] : Internal Error !
2>Hybridizer out: [DONE]
2>Done building project "HelloWorld_CUDA.vs2017.vcxproj" -- FAILED.
========== Rebuild All: 1 succeeded, 1 failed, 0 skipped ==========
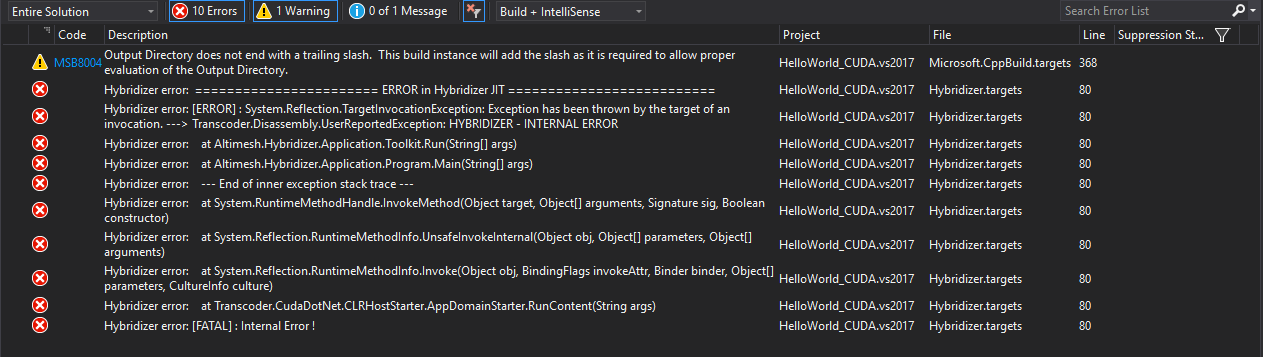