First point of the curve = last point of the previous curve?
Help to solve it. Thanks a lot.
Here:
CanvasPolyLine.prototype.bezierCurveTo = function bezierCurveTo (x1, y1, x2, y2, x, y) {
var this$1 = this;
var points = adaptiveBezierCurve(
[this._x0, this._y0],
[x1, y1],
[x2, y2],
[this._x0 = this._x1 = +x, this._y0 = this._y1 = +y],
+this._curveScale
);
points.forEach(function (p) {
this$1.lineTo(p[0], p[1]);
});
};
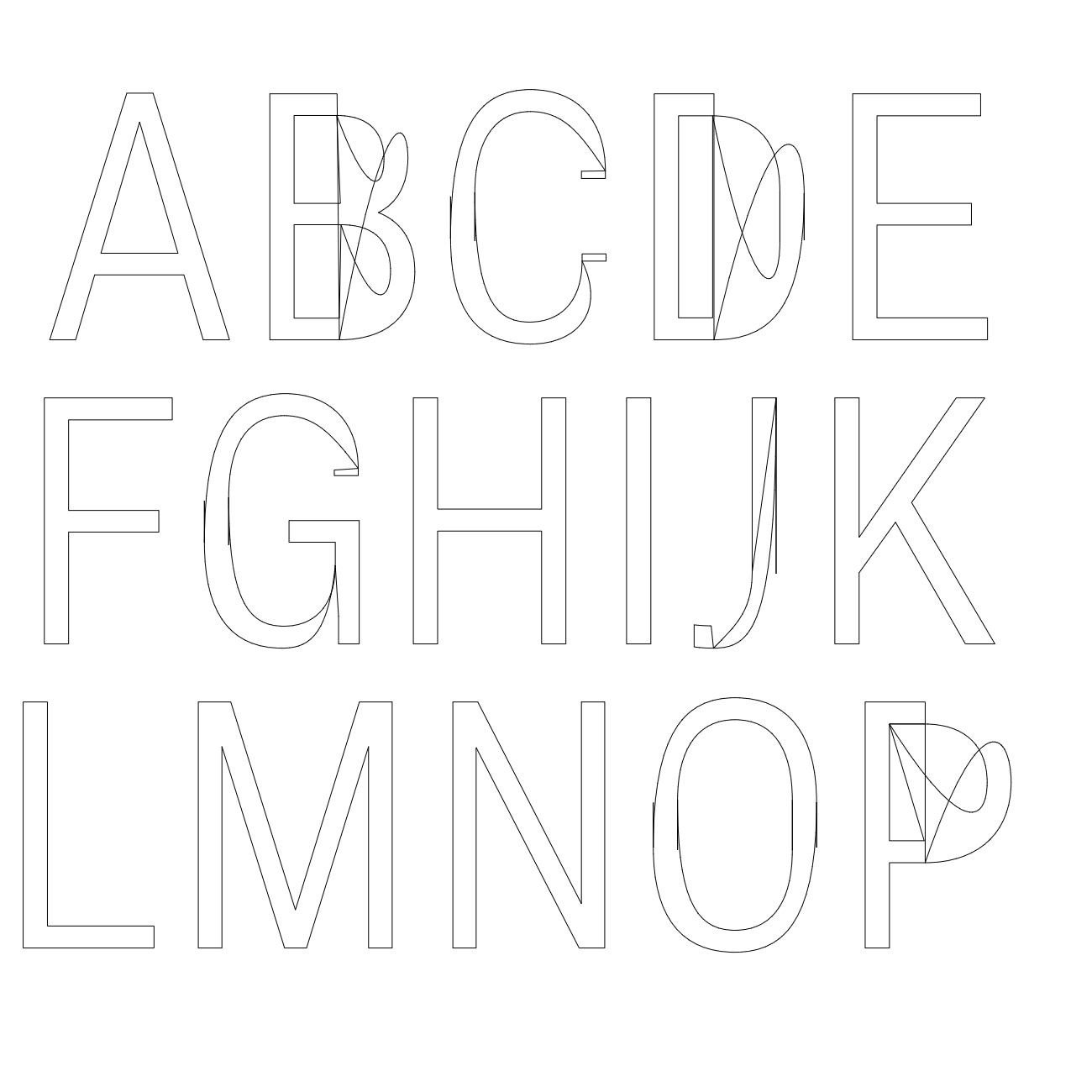
My canvas:
ctx.save(); ctx.strokeStyle="rgba(0,0,0,0)"; ctx.miterLimit=4; ctx.font="normal normal 400 normal 15px / 21.4286px ''"; ctx.font=" 15px "; ctx.scale(1,1); ctx.save(); ctx.fillStyle="#121313"; ctx.font=" 15px "; ctx.beginPath(); ctx.moveTo(1066.1,621.684); ctx.lineTo(1022.7599999999999,681.8209999999999); ctx.lineTo(1022.7599999999999,766.56); ctx.lineTo(993.8599999999999,766.56); ctx.lineTo(993.8599999999999,473.684); ctx.lineTo(1022.7599999999999,473.684); ctx.lineTo(1022.7599999999999,639.647); ctx.lineTo(1138.35,473.6840000000001); ctx.lineTo(1172.32,473.6840000000001); ctx.lineTo(1085.24,598.2540000000001); ctx.lineTo(1184.43,766.5600000000002); ctx.lineTo(1149.28,766.5600000000002); ctx.closePath(); ctx.moveTo(1015.1299999999999,111.68399999999997); ctx.lineTo(1167.4299999999998,111.68399999999997); ctx.lineTo(1167.4299999999998,137.84799999999996); ctx.lineTo(1044.0299999999997,137.84799999999996); ctx.lineTo(1044.0299999999997,242.112); ctx.lineTo(1156.4899999999998,242.112); ctx.lineTo(1156.4899999999998,267.885); ctx.lineTo(1044.03,267.885); ctx.lineTo(1044.03,378.4); ctx.lineTo(1175.6299999999999,378.4); ctx.lineTo(1175.6299999999999,404.56); ctx.lineTo(1015.1299999999999,404.56); ctx.lineTo(1015.1299999999999,111.684); ctx.closePath(); ctx.moveTo(874.934,1133.64); ctx.bezierCurveTo(811.2819999999999,1133.64,777.699,1091.8500000000001,777.699,1008.6800000000001); ctx.lineTo(777.699,955.568); ctx.bezierCurveTo(777.699,872.7819999999999,814.406,830.608,874.934,830.608); ctx.bezierCurveTo(938.586,830.608,972.169,873.563,972.169,955.568); ctx.lineTo(972.169,1008.68); ctx.bezierCurveTo(972.169,1091.46,936.243,1133.64,874.934,1133.64); ctx.closePath(); ctx.moveTo(943.2719999999999,952.835); ctx.bezierCurveTo(943.2719999999999,890.355,919.842,856.7710000000001,874.934,856.7710000000001); ctx.bezierCurveTo(830.807,856.7710000000001,806.596,891.1360000000001,806.596,952.835); ctx.lineTo(806.596,1011.8); ctx.bezierCurveTo(806.596,1074.28,830.417,1107.47,874.934,1107.47); ctx.bezierCurveTo(919.4509999999999,1107.47,943.2719999999999,1073.5,943.2719999999999,1011.8000000000001); ctx.lineTo(943.2719999999999,952.835); ctx.closePath(); ctx.moveTo(923.967,473.684); ctx.lineTo(923.967,682.6); ctx.bezierCurveTo(923.967,743.518,896.632,771.634,849.382,771.634); ctx.translate(847.4638989736868,629.2219165468996); ctx.rotate(0); ctx.arc(0,0,142.425,1.557328473449509,1.719647296429975,0); ctx.rotate(0); ctx.translate(-847.4638989736868,-629.2219165468996); ctx.lineTo(826.342,743.911); ctx.translate(847.2834371978728,557.4170757984658); ctx.rotate(0); ctx.arc(0,0,187.666,1.6826180914012192,1.5741823339144265,1); ctx.rotate(0); ctx.translate(-847.2834371978728,-557.4170757984658); ctx.bezierCurveTo(875.936,745.082,895.461,727.119,895.461,681.04); ctx.lineTo(895.461,473.684); ctx.lineTo(923.967,473.684); ctx.closePath(); ctx.moveTo(849.956,404.56); ctx.lineTo(778.884,404.56); ctx.lineTo(778.884,111.684); ctx.lineTo(849.956,111.684); ctx.bezierCurveTo(917.1220000000001,111.684,957.3430000000001,148.001,957.3430000000001,230.397); ctx.lineTo(957.3430000000001,285.848); ctx.bezierCurveTo(957.343,367.072,917.512,404.56,849.956,404.56); ctx.closePath(); ctx.moveTo(928.446,228.054); ctx.bezierCurveTo(928.446,164.793,897.9870000000001,137.848,848.394,137.848); ctx.lineTo(807.781,137.848); ctx.lineTo(807.781,378.4); ctx.lineTo(848.394,378.4); ctx.bezierCurveTo(897.594,378.4,928.446,351.065,928.446,288.195); ctx.lineTo(928.446,228.054); ctx.closePath(); ctx.moveTo(745.9,473.684); ctx.lineTo(774.8,473.684); ctx.lineTo(774.8,766.56); ctx.lineTo(745.9,766.56); ctx.lineTo(745.9,473.684); ctx.closePath(); ctx.moveTo(631.28,409.636); ctx.bezierCurveTo(568.8,409.636,536.389,370.586,536.389,283.505); ctx.lineTo(536.389,233.911); ctx.bezierCurveTo(536.389,152.687,569.189,106.611,631.28,106.611); ctx.bezierCurveTo(690.246,106.611,720.7049999999999,146.051,720.7049999999999,203.45499999999998); ctx.lineTo(720.7049999999999,212.43699999999998); ctx.lineTo(692.2,212.43699999999998); ctx.lineTo(692.2,203.84599999999998); ctx.bezierCurveTo(692.2,160.49999999999997,671.894,132.77399999999997,631.282,132.77399999999997); ctx.bezierCurveTo(588.7180000000001,132.77399999999997,565.288,167.91999999999996,565.288,229.61899999999997); ctx.lineTo(565.288,287.01899999999995); ctx.bezierCurveTo(565.288,351.0609999999999,587.546,383.47299999999996,630.111,383.47299999999996); ctx.bezierCurveTo(669.942,383.47299999999996,692.981,356.138,692.981,310.84); ctx.lineTo(692.981,302.24899999999997); ctx.lineTo(721.4879999999999,302.24899999999997); ctx.lineTo(721.4879999999999,310.84); ctx.bezierCurveTo(721.487,369.805,688.294,409.636,631.281,409.636); ctx.closePath(); ctx.moveTo(644.766,473.684); ctx.lineTo(673.6659999999999,473.684); ctx.lineTo(673.6659999999999,766.56); ctx.lineTo(644.766,766.56); ctx.lineTo(644.766,632.228); ctx.lineTo(520.978,632.228); ctx.lineTo(520.978,766.56); ctx.lineTo(492.078,766.56); ctx.lineTo(492.078,473.684); ctx.lineTo(520.978,473.684); ctx.lineTo(520.978,606.0640000000001); ctx.lineTo(644.767,606.0640000000001); ctx.lineTo(644.767,473.684); ctx.closePath(); ctx.moveTo(493.826,323.336); ctx.bezierCurveTo(493.826,372.536,463.75800000000004,404.56,404.011,404.56); ctx.lineTo(321.225,404.56); ctx.lineTo(321.225,111.684); ctx.lineTo(401.278,111.684); ctx.bezierCurveTo(456.72900000000004,111.684,485.62600000000003,140.972,485.62600000000003,188.223); ctx.bezierCurveTo(485.62600000000003,220.24400000000003,472.34900000000005,242.502,450.48100000000005,253.046); ctx.bezierCurveTo(478.206,263.98,493.826,287.8,493.826,323.336); ctx.closePath(); ctx.moveTo(457.119,189.785); ctx.bezierCurveTo(457.119,155.42,437.204,137.457,400.88700000000006,137.457); ctx.lineTo(350.122,137.457); ctx.lineTo(350.122,242.112); ctx.lineTo(405.183,242.112); ctx.bezierCurveTo(439.547,242.112,457.119,222.2,457.119,189.785); ctx.closePath(); ctx.moveTo(405.96400000000006,267.494); ctx.lineTo(350.122,267.494); ctx.lineTo(350.122,378.787); ctx.lineTo(404.011,378.787); ctx.bezierCurveTo(442.67100000000005,378.787,464.92900000000003,358.087,464.92900000000003,322.945); ctx.bezierCurveTo(464.929,287.019,445.4,267.494,405.964,267.494); ctx.closePath(); ctx.moveTo(426.48,557.642); ctx.lineTo(426.48,566.2330000000001); ctx.lineTo(397.974,566.2330000000001); ctx.lineTo(397.974,559.6); ctx.bezierCurveTo(397.974,520.159,376.887,494.776,338.227,494.776); ctx.bezierCurveTo(295.272,494.776,272.23299999999995,528.75,272.23299999999995,592.011); ctx.lineTo(272.23299999999995,648.634); ctx.bezierCurveTo(272.23299999999995,713.066,294.49099999999993,745.4780000000001,337.83299999999997,745.4780000000001); ctx.bezierCurveTo(376.883,745.4780000000001,399.14099999999996,718.533,399.14099999999996,673.2350000000001); ctx.lineTo(399.14099999999996,645.505); ctx.lineTo(344.08099999999996,645.505); ctx.lineTo(344.08099999999996,619.732); ctx.lineTo(427.64799999999997,619.732); ctx.lineTo(427.64799999999997,766.56); ctx.lineTo(403.04799999999994,766.56); ctx.lineTo(403.04799999999994,733.367); ctx.bezierCurveTo(390.54799999999994,757.578,367.5129999999999,771.636,335.88199999999995,771.636); ctx.bezierCurveTo(274.96399999999994,771.636,243.33399999999995,731.415,243.33399999999995,645.895); ctx.lineTo(243.33399999999995,596.3); ctx.bezierCurveTo(243.33399999999995,513.9,276.9169999999999,468.60599999999994,339.39699999999993,468.60599999999994); ctx.bezierCurveTo(394.85,468.608,426.48,504.534,426.48,557.642); ctx.closePath(); ctx.moveTo(218.91700000000003,327.2420000000001); ctx.lineTo(112.7,327.2420000000001); ctx.lineTo(90.051,404.56); ctx.lineTo(59.2,404.56); ctx.lineTo(150.969,110.9); ctx.lineTo(182.209,110.9); ctx.lineTo(273.2,404.56); ctx.lineTo(241.95999999999998,404.56); ctx.closePath(); ctx.moveTo(166.2,145.267); ctx.lineTo(120.12099999999998,301.467); ctx.lineTo(211.88899999999998,301.467); ctx.closePath(); ctx.moveTo(205.07,499.848); ctx.lineTo(81.671,499.848); ctx.lineTo(81.671,607.626); ctx.lineTo(189.059,607.626); ctx.lineTo(189.059,633.4); ctx.lineTo(81.671,633.4); ctx.lineTo(81.671,766.56); ctx.lineTo(52.77100000000001,766.56); ctx.lineTo(52.77100000000001,473.684); ctx.lineTo(205.069,473.684); ctx.lineTo(205.069,499.848); ctx.closePath(); ctx.moveTo(56.457,1102.4); ctx.lineTo(183.37,1102.4); ctx.lineTo(183.37,1128.5600000000002); ctx.lineTo(27.56,1128.5600000000002); ctx.lineTo(27.56,835.684); ctx.lineTo(56.459999999999994,835.684); ctx.lineTo(56.459999999999994,1102.4); ctx.closePath(); ctx.moveTo(351.671,1083.26); ctx.lineTo(427.81899999999996,835.684); ctx.lineTo(466.86899999999997,835.684); ctx.lineTo(466.86899999999997,1128.56); ctx.lineTo(438.753,1128.56); ctx.lineTo(438.753,888.793); ctx.lineTo(364.948,1128.56); ctx.lineTo(338.785,1128.56); ctx.lineTo(264.2,888.793); ctx.lineTo(264.2,1128.56); ctx.lineTo(236.083,1128.56); ctx.lineTo(236.083,835.684); ctx.lineTo(274.743,835.684); ctx.closePath(); ctx.moveTo(692.185,1075.84); ctx.lineTo(692.185,835.684); ctx.lineTo(719.91,835.684); ctx.lineTo(719.91,1128.56); ctx.lineTo(689.451,1128.56); ctx.lineTo(566.8340000000001,889.9599999999999); ctx.lineTo(566.8340000000001,1128.56); ctx.lineTo(539.109,1128.56); ctx.lineTo(539.109,835.684); ctx.lineTo(568.787,835.684); ctx.closePath(); ctx.moveTo(1203.73,931.357); ctx.bezierCurveTo(1203.73,993.057,1162.73,1027.03,1101.42,1027.03); ctx.lineTo(1058.8500000000001,1027.03); ctx.lineTo(1058.8500000000001,1128.56); ctx.lineTo(1029.96,1128.56); ctx.lineTo(1029.96,835.684); ctx.lineTo(1101.42,835.684); ctx.bezierCurveTo(1165.85,835.684,1203.73,870.439,1203.73,931.357); ctx.closePath(); ctx.moveTo(1058.85,861.848); ctx.lineTo(1058.85,1000.87); ctx.lineTo(1100.25,1000.87); ctx.bezierCurveTo(1146.33,1000.87,1175.22,977.046,1175.22,931.357); ctx.bezierCurveTo(1175.22,886.057,1146.72,861.848,1100.25,861.848); ctx.lineTo(1058.85,861.848); ctx.closePath(); ctx.restore(); ctx.restore(); canvasCtx.stroke()