Please provide us with the following information:
This issue is for a: (mark with an x
)
- [X] bug report -> please search issues before submitting
- [ ] feature request
- [ ] documentation issue or request
- [ ] regression (a behavior that used to work and stopped in a new release)
Minimal steps to reproduce
Running the bot from node.js sending the bot a message in slack or even allow it to sit prompts it to start spewing responses from QnA Maker. It works fine in my Teams channel, but keeps doing this behavior in Slack.
Any log messages given by the failure
none
Expected/desired behavior
Not to repeat itself
OS and Version?
Windows 7, 8 or 10. Linux (which distribution). macOS (Yosemite? El Capitan? Sierra?) Windows 10
Versions
botkit
Mention any other details that might be useful
see attached screenshots
Thanks! We'll be in touch soon.
`require('dotenv').config();
const express = require("express");
const bodyParser = require("body-parser");
// Import Botkit's core features
const { Botkit } = require("botkit");
// Import a platform-specific adapter for slack.
const { SlackAdapter, SlackEventMiddleware, SlackMessageTypeMiddleware } = require('botbuilder-adapter-slack');
const { BotFrameworkAdapter } = require('botbuilder');
const { TwilioAdapter } = require('botbuilder-adapter-twilio-sms');
const { QnAMaker } = require('botbuilder-ai');
// Create an express server with json and urlencoded body parsing
const webserver = express();
webserver.use((req, res, next) => {
req.rawBody = '';
req.on('data', function (chunk) {
req.rawBody += chunk;
});
next();
});
webserver.use(express.json());
webserver.use(express.urlencoded({ extended: true }));
//Create Bot Framework Adapter.
// See https://aka.ms/about-bot-adapter to learn more about adapters and how bots work.
const botAdapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
// Adaptor for Slack
const slackAdaptor = new SlackAdapter({
// REMOVE THIS OPTION AFTER YOU HAVE CONFIGURED YOUR APP!
//enable_incomplete: true,
// The following options are sufficient for a single team application
// parameters used to secure webhook endpoint
verificationToken: process.env.SLACK_VERIFICATION_TOKEN,
clientSigningSecret: process.env.SLACK_CLIENT_SIGNING_SECRET,
// auth token for a single-team app
botToken: process.env.SLACK_BOT_TOKEN
});
// Use Slack Middleware to emit events that match their original Slack event types.
//slackAdaptor.use(new SlackMessageTypeMiddleware());
//slackAdaptor.use(new SlackEventMiddleware());
// Controller for Slack
const slackController = new Botkit({
webhook_uri: process.env.SLACK_WEBHOOK_URI,
adapter: slackAdaptor,
webserver: webserver
});
// Controller for Teams
const teamsController = new Botkit({
webhook_uri: process.env.TEAMS_WEBHOOK_URI,
adapterConfig: {
appId: process.env.TEAMS_APP_ID,
appPassword: process.env.TEAMS_APP_PASSWORD,
},
webserver: webserver
});
// QnA Integration
const qnaMaker = new QnAMaker({
knowledgeBaseId: process.env.QnAKnowledgebaseId,
endpointKey: process.env.QnAAuthKey,
host: process.env.QnAEndpointHostName
});
// QnA Integration for Teams
teamsController.middleware.ingest.use(async (bot, message, next) => {
if (message.incoming_message.type === 'message') {
const qnaResults = await qnaMaker.getAnswers(message.context);
if (qnaResults[0]) {
// If we got an answer, send it directly
//await message.context.sendActivity(qnaResults[0].answer);
await bot.reply(message, qnaResults[0].answer); // also works
} else {
// If we have no other features, we could just say we didn't find any answers:
//await message.context.sendActivity('No QnA Maker answers were found.');
// Otherwise, just forward to the next BotHandler to see if there are any other matches
next();
}
} else {
next();
}
});
// QnA Integration for Slack
slackController.middleware.ingest.use(async (bot, message, next) => {
if (message.incoming_message.type === 'message') {
const qnaResults = await qnaMaker.getAnswers(message.context);
if (qnaResults[0]) {
// If we got an answer, send it directly
//await message.context.sendActivity(qnaResults[0].answer);
await bot.reply(message, qnaResults[0].answer); // also works
} else {
// If we have no other features, we could just say we didn't find any answers:
await message.context.sendActivity('No QnA Maker answers were found.');
// Otherwise, just forward to the next BotHandler to see if there are any other matches
next();
}
} else {
slackAdaptor.use(new SlackMessageTypeMiddleware());
slackAdaptor.use(new SlackEventMiddleware());
}
});
//Adapter and Controller for Twilio
const smsadapter = new TwilioAdapter({
twilio_number: process.env.TWILIO_NUMBER,
account_sid: process.env.TWILIO_ACCOUNT_SID,
auth_token: process.env.TWILIO_AUTH_TOKEN,
});
const smsController = new Botkit({
adapter: smsadapter,
webserver: webserver
});
// Catch-all for errors.
botAdapter.onTurnError = async (context, error) => {
// This check writes out errors to console log
// NOTE: In production environment, you should consider logging this to Azure
// application insights.
console.error(\n [onTurnError]: ${ error }
);
// Send a message to the user
await context.sendActivity(Oops. Something went wrong!
);
// Clear out state
await conversationState.delete(context);
};
// load developer-created local custom feature modules.
// Load common features for slack and teams
slackController.loadModules(__dirname + '/features/common');
teamsController.loadModules(__dirname + '/features/common');
// Load platform specific features seperately
slackController.loadModules(__dirname + '/features/slack');
teamsController.loadModules(__dirname + '/features/teams');
smsController.loadModules(__dirname + '/features/twilio');
// For running server locally for development purposes
if (require.main === module) {
const http = require("http");
const port = process.env.PORT || 3000;
const httpserver = http.createServer(webserver);
httpserver.listen(port, function () {
console.log("Slack Webhook endpoint online: http://127.0.0.1:" + port + process.env.SLACK_WEBHOOK_URI);
console.log("Teams Webhook endpoint online: http://127.0.0.1:" + port + process.env.TEAMS_WEBHOOK_URI);
});
};`
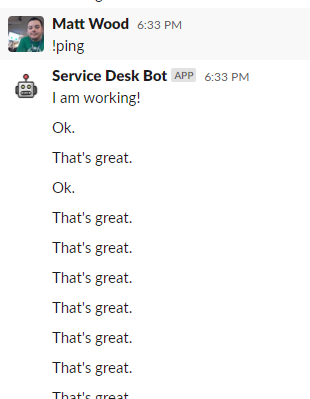